Assembly Language programming : 8086 Assembler Tutorial (Part 11)
Making your own Operating System
Usually, when a computer starts it will try to load the first 512-byte sector (that's Cylinder 0, Head 0, Sector 1) from any diskette in your A: drive to memory location 0000h:7C00h and give it control. If this fails, the BIOS tries to use the MBR of the first hard drive instead.
This tutorial covers booting up from a floppy drive, the same principles are used to boot from a hard drive. But using a floppy drive has several advantages:
Example of a simple floppy disk boot program:
Copy the above example to Emu8086 source editor and press[Compile
and Emulate] button. The Emulator automatically loads ".boot"
file to 0000h:7C00h.
You can run it just like a regular program, or you can use the Virtual Drive menu to Write 512 bytes at 7C00h to the Boot Sector of a virtual floppy drive (FLOPPY_0 file in Emulator's folder).
After writing your program to the Virtual Floppy Drive, you can select Boot from Floppy from Virtual Drive menu.
If you are curious, you may write the virtual floppy (FLOPPY_0) or ".boot" file to a real floppy disk and boot your computer from it, I recommend using "RawWrite for Windows" from: http://www.chrysocome.net/rawwrite
(recent builds now work under all versions of Windows!)
Note: however, that this .boot file is not an MS-DOS compatible boot sector (it will not allow you to read or write data on this diskette until you format it again), so don't bother writing only this sector to a diskette with data on it. As a matter of fact, if you use any 'raw-write' programs, such at the one listed above, they will erase all of the data anyway. So make sure the diskette you use doesn't contain any important data.
".boot" files are limited to 512 bytes (sector size). If your new Operating System is going to grow over this size, you will need to use a boot program to load data from other sectors. A good example of a tiny Operating System can be found in "Samples" folder as:
micro-os_loader.asm
micro-os_kernel.asm
To create extensions for your Operating System (over 512 bytes), you can use ".bin" files (select "BIN Template" from "File" -> "New" menu).
To write ".bin" file to virtual floppy, select "Write .bin file to floppy..." from "Virtual Drive" menu of emulator:
You can also use this to write ".boot" files.
Idealized floppy drive and diskette structure:
For a 1440 kb diskette:
Assembly Language : 8086 Assembler Tutorial Part 12
Assembly Language : 8086 Assembler Tutorial Part 11
Assembly Language : 8086 Assembler Tutorial Part 10
Assembly Language : 8086 Assembler Tutorial Part 9
Assembly Language : 8086 Assembler Tutorial Part 8
Assembly Language : 8086 Assembler Tutorial Part 7
Assembly Language : 8086 Assembler Tutorial Part 6
Assembly Language : 8086 Assembler Tutorial Part 5
Assembly Language : 8086 Assembler Tutorial Part 4
Assembly Language : 8086 Assembler Tutorial Part 3
Assembly Language : 8086 Assembler Tutorial Part 2
Assembly Language : 8086 Assembler Tutorial Part 1
Assembly Language Programming : Complete 8086 instruction sets
Assembly Language Programming : I/O ports - IN/OUT instructions
Assembly Language programming : Emu8086 Assembler Compiling and MASM / TASM compatibility
Assembly Language - string convert - Lowercase , Uppercase
for programming : the language of Number
Assembly Language - Complete Instruction Set and Instruction Timing of 8086 microprocessors
Assembly Language programming : A list of emulator supported interrupts
Assembly Language Programming : Emu8086 Overview, Using Emulator, Virtual Drives
Assembly Language Programming : All about Memory - Global Memory Table and Custom Memory Map
buy me a cup of coffee
buy me a cup of coffee
Need More Detail ? contact me !!
Send me any small amount of money is welcome.
___________________________________________
Don't know how to send money ? Click here for detail about Paypal account.
About PayPal Payment Methods
What type of PayPal accounts is better.
Don't have money? OK! Here is another way to get the program.
how to get my program - Free of charge
Making your own Operating System
Usually, when a computer starts it will try to load the first 512-byte sector (that's Cylinder 0, Head 0, Sector 1) from any diskette in your A: drive to memory location 0000h:7C00h and give it control. If this fails, the BIOS tries to use the MBR of the first hard drive instead.
This tutorial covers booting up from a floppy drive, the same principles are used to boot from a hard drive. But using a floppy drive has several advantages:
- You can keep your existing operating system intact (Windows, DOS...).
- It is easy to modify the boot record of a floppy disk.
Example of a simple floppy disk boot program:
; directive to create BOOT file:
#MAKE_BOOT#
; Boot record is loaded at 0000:7C00,
; so inform compiler to make required
; corrections:
ORG 7C00h
; load message address into SI register:
LEA SI, msg
; teletype function id:
MOV AH, 0Eh
print: MOV AL, [SI]
CMP AL, 0
JZ done
INT 10h ; print using teletype.
INC SI
JMP print
; wait for 'any key':
done: MOV AH, 0
INT 16h
; store magic value at 0040h:0072h:
; 0000h - cold boot.
; 1234h - warm boot.
MOV AX, 0040h
MOV DS, AX
MOV w.[0072h], 0000h ; cold boot.
JMP 0FFFFh:0000h ; reboot!
new_line EQU 13, 10
msg DB 'Hello This is My First Boot Program!'
DB new_line, 'Press any key to reboot', 0
|
Copy the above example to Emu8086 source editor and press
You can run it just like a regular program, or you can use the Virtual Drive menu to Write 512 bytes at 7C00h to the Boot Sector of a virtual floppy drive (FLOPPY_0 file in Emulator's folder).
After writing your program to the Virtual Floppy Drive, you can select Boot from Floppy from Virtual Drive menu.
If you are curious, you may write the virtual floppy (FLOPPY_0) or ".boot" file to a real floppy disk and boot your computer from it, I recommend using "RawWrite for Windows" from: http://www.chrysocome.net/rawwrite
(recent builds now work under all versions of Windows!)
Note: however, that this .boot file is not an MS-DOS compatible boot sector (it will not allow you to read or write data on this diskette until you format it again), so don't bother writing only this sector to a diskette with data on it. As a matter of fact, if you use any 'raw-write' programs, such at the one listed above, they will erase all of the data anyway. So make sure the diskette you use doesn't contain any important data.
".boot" files are limited to 512 bytes (sector size). If your new Operating System is going to grow over this size, you will need to use a boot program to load data from other sectors. A good example of a tiny Operating System can be found in "Samples" folder as:
micro-os_loader.asm
micro-os_kernel.asm
To create extensions for your Operating System (over 512 bytes), you can use ".bin" files (select "BIN Template" from "File" -> "New" menu).
To write ".bin" file to virtual floppy, select "Write .bin file to floppy..." from "Virtual Drive" menu of emulator:
You can also use this to write ".boot" files.
Sector at:Cylinder: 0is the boot sector! |
Idealized floppy drive and diskette structure:
For a 1440 kb diskette:
- Floppy disk has 2 sides, and there are 2 heads; one for each side
(0..1), the drive heads move above the surface of the disk on
each side.
- Each side has 80 cylinders (numbered 0..79).
- Each cylinder has 18 sectors (1..18).
- Each sector has 512 bytes.
- Total size of floppy disk is: 2 x 80 x 18 x 512 = 1,474,560 bytes.
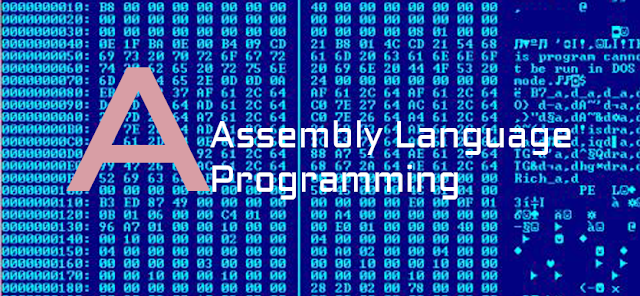
emu8086 is better than NASM, MASM or TASM
Tag: 8086 Assembler, 8086 microprocessors instruction, assembly code, Assembly coding, assembly guide, assembly instruction, assembly language, assembly language instruction set, assembly language programming, Assembly program, assembly programming, capital letter, character convert, complete 8086 instruction sets microprocessors, complete instruction timing and instruction sets for 8086 microprocessors, conversion of characters in assembly language programming 8086, convert, emu8086, instruction complete set, instruction set complete for 8086, instruction sets, instruction sets for 8086, Lower case, Lowercase, print the small character into capital letter, programming 8086 assembly language conversion of small characters to capital, small letter, text string convert, Tutorial,Assembly Language : 8086 Assembler Tutorial Part 12
Assembly Language : 8086 Assembler Tutorial Part 11
Assembly Language : 8086 Assembler Tutorial Part 10
Assembly Language : 8086 Assembler Tutorial Part 9
Assembly Language : 8086 Assembler Tutorial Part 8
Assembly Language : 8086 Assembler Tutorial Part 7
Assembly Language : 8086 Assembler Tutorial Part 6
Assembly Language : 8086 Assembler Tutorial Part 5
Assembly Language : 8086 Assembler Tutorial Part 4
Assembly Language : 8086 Assembler Tutorial Part 3
Assembly Language : 8086 Assembler Tutorial Part 2
Assembly Language : 8086 Assembler Tutorial Part 1
Assembly Language Programming : Complete 8086 instruction sets
Assembly Language Programming : I/O ports - IN/OUT instructions
Assembly Language programming : Emu8086 Assembler Compiling and MASM / TASM compatibility
Assembly Language - string convert - Lowercase , Uppercase
for programming : the language of Number
Assembly Language - Complete Instruction Set and Instruction Timing of 8086 microprocessors
Assembly Language programming : A list of emulator supported interrupts
Assembly Language Programming : Emu8086 Overview, Using Emulator, Virtual Drives
Assembly Language Programming : All about Memory - Global Memory Table and Custom Memory Map
buy me a cup of coffee
My Paypal Account is : ksw.industries@gmail.com
Send me any small amount of money is welcome.buy me a cup of coffee
___________________________________________
Need More Detail ? contact me !!
My Paypal Account is : ksw.industries@gmail.com
buy me a cup of coffeeSend me any small amount of money is welcome.
___________________________________________
Don't know how to send money ? Click here for detail about Paypal account.
About PayPal Payment Methods
What type of PayPal accounts is better.
Don't have money? OK! Here is another way to get the program.
how to get my program - Free of charge