Assembly Language programming : 8086 Assembler Tutorial (Part 2)
Memory Access
To access memory we can use these four registers: BX, SI, DI, BP.
Combining these registers inside [ ] symbols, we can get different memory locations. These combinations are supported (addressing modes):
d8 - stays for 8 bit displacement.
d16 - stays for 16 bit displacement.
Displacement can be a immediate value or offset of a variable, or even both. It's up to compiler to calculate a single immediate value.
Displacement can be inside or outside of [ ] symbols, compiler generates the same machine code for both ways.
Displacement is a signed value, so it can be both positive or negative.
Generally the compiler takes care about difference between d8 and d16, and generates the required machine code.
For example, let's assume that DS = 100, BX = 30, SI = 70.
The following addressing mode: [BX + SI] + 25
is calculated by processor to this physical address:100 * 16 + 30 + 70 + 25 = 1725.
By default DS segment register is used for all modes except those with BP register, for these SS segment register is used.
There is an easy way to remember all those possible combinations using this chart:
You can form all valid combinations by taking only one item from each column or skipping the column by not taking anything from it. As you see BX and BP never go together. SI and DI also don't go together. Here is an example of a valid addressing mode: [BX+5].
The value in segment register (CS, DS, SS, ES) is called a "segment",
and the value in purpose register (BX, SI, DI, BP) is called an "offset".
When DS contains value 1234h and SI contains the value 7890h it can be also recorded as 1234:7890. The physical address will be 1234h * 10h + 7890h = 19BD0h.
In order to say the compiler about data type,
these prefixes should be used:
BYTE PTR - for byte.
WORD PTR - for word (two bytes).
For example:
b. - for BYTE PTR
w. - for WORD PTR
sometimes compiler can calculate the data type automatically, but you may not and should not rely on that when one of the operands is an immediate value.
MOV instruction
The MOV instruction cannot be used to set the value of the CS and IP registers.
You can copy & paste the above program to Emu8086 code editor, and press [Compile and Emulate] button (or press F5 key on your keyboard).
The Emulator window should open with this program loaded, click[Single Step]
button and watch the register values.
How to do copy & paste:
As you may guess, ";" is used for comments, anything after ";" symbol is ignored by compiler.
You should see something like that when program finishes:
Actually the above program writes directly to video memory, so you may see that MOV is a very powerful instruction.
Assembly Language : 8086 Assembler Tutorial Part 12
Assembly Language : 8086 Assembler Tutorial Part 11
Assembly Language : 8086 Assembler Tutorial Part 10
Assembly Language : 8086 Assembler Tutorial Part 9
Assembly Language : 8086 Assembler Tutorial Part 8
Assembly Language : 8086 Assembler Tutorial Part 7
Assembly Language : 8086 Assembler Tutorial Part 6
Assembly Language : 8086 Assembler Tutorial Part 5
Assembly Language : 8086 Assembler Tutorial Part 4
Assembly Language : 8086 Assembler Tutorial Part 3
Assembly Language : 8086 Assembler Tutorial Part 2
Assembly Language : 8086 Assembler Tutorial Part 1
Assembly Language Programming : Complete 8086 instruction sets
Assembly Language Programming : I/O ports - IN/OUT instructions
Assembly Language programming : Emu8086 Assembler Compiling and MASM / TASM compatibility
Assembly Language - string convert - Lowercase , Uppercase
for programming : the language of Number
Assembly Language - Complete Instruction Set and Instruction Timing of 8086 microprocessors
Assembly Language programming : A list of emulator supported interrupts
Assembly Language Programming : Emu8086 Overview, Using Emulator, Virtual Drives
Assembly Language Programming : All about Memory - Global Memory Table and Custom Memory Map
buy me a cup of coffee
buy me a cup of coffee
Need More Detail ? contact me !!
Send me any small amount of money is welcome.
___________________________________________
Don't know how to send money ? Click here for detail about Paypal account.
About PayPal Payment Methods
What type of PayPal accounts is better.
Don't have money? OK! Here is another way to get the program.
how to get my program - Free of charge
Memory Access
To access memory we can use these four registers: BX, SI, DI, BP.
Combining these registers inside [ ] symbols, we can get different memory locations. These combinations are supported (addressing modes):
[BX + SI] [BX + DI] [BP + SI] [BP + DI] |
[SI] [DI] d16 (variable offset only) [BX] |
[BX + SI] + d8 [BX + DI] + d8 [BP + SI] + d8 [BP + DI] + d8 |
[SI] + d8 [DI] + d8 [BP] + d8 [BX] + d8 |
[BX + SI] + d16 [BX + DI] + d16 [BP + SI] + d16 [BP + DI] + d16 |
[SI] + d16 [DI] + d16 [BP] + d16 [BX] + d16 |
d8 - stays for 8 bit displacement.
d16 - stays for 16 bit displacement.
Displacement can be a immediate value or offset of a variable, or even both. It's up to compiler to calculate a single immediate value.
Displacement can be inside or outside of [ ] symbols, compiler generates the same machine code for both ways.
Displacement is a signed value, so it can be both positive or negative.
Generally the compiler takes care about difference between d8 and d16, and generates the required machine code.
For example, let's assume that DS = 100, BX = 30, SI = 70.
The following addressing mode: [BX + SI] + 25
is calculated by processor to this physical address:
By default DS segment register is used for all modes except those with BP register, for these SS segment register is used.
There is an easy way to remember all those possible combinations using this chart:
You can form all valid combinations by taking only one item from each column or skipping the column by not taking anything from it. As you see BX and BP never go together. SI and DI also don't go together. Here is an example of a valid addressing mode: [BX+5].
The value in segment register (CS, DS, SS, ES) is called a "segment",
and the value in purpose register (BX, SI, DI, BP) is called an "offset".
When DS contains value 1234h and SI contains the value 7890h it can be also recorded as 1234:7890. The physical address will be 1234h * 10h + 7890h = 19BD0h.
In order to say the compiler about data type,
these prefixes should be used:
BYTE PTR - for byte.
WORD PTR - for word (two bytes).
For example:
BYTE PTR [BX] ; byte access.
or
WORD PTR [BX] ; word access.
Emu8086 supports shorter prefixes as well:b. - for BYTE PTR
w. - for WORD PTR
sometimes compiler can calculate the data type automatically, but you may not and should not rely on that when one of the operands is an immediate value.
MOV instruction
-
Copies the second operand (source) to the first
operand (destination).
-
The source operand can be an immediate value,
general-purpose register or memory location.
-
The destination register can be a general-purpose register, or memory location.
- Both operands must be the same size, which can be a byte or a word.
These types of operands are supported:MOV REG, memoryREG: AX, BX, CX, DX, AH, AL, BL, BH, CH, CL, DH, DL, DI, SI, BP, SP. memory: [BX], [BX+SI+7], variable, etc... immediate: 5, -24, 3Fh, 10001101b, etc... |
For segment registers only these types of MOV are supported:MOV SREG, memorySREG: DS, ES, SS, and only as second operand: CS. REG: AX, BX, CX, DX, AH, AL, BL, BH, CH, CL, DH, DL, DI, SI, BP, SP. memory: [BX], [BX+SI+7], variable, etc... |
The MOV instruction cannot be used to set the value of the CS and IP registers.
Here is a short program that demonstrates the use of MOV instruction:
#MAKE_COM# ; instruct compiler to make COM file.
ORG 100h ; directive required for a COM program.
MOV AX, 0B800h ; set AX to hexadecimal value of B800h.
MOV DS, AX ; copy value of AX to DS.
MOV CL, 'A' ; set CL to ASCII code of 'A', it is 41h.
MOV CH, 01011111b ; set CH to binary value.
MOV BX, 15Eh ; set BX to 15Eh.
MOV [BX], CX ; copy contents of CX to memory at B800:015E
RET ; returns to operating system.
|
You can copy & paste the above program to Emu8086 code editor, and press [Compile and Emulate] button (or press F5 key on your keyboard).
The Emulator window should open with this program loaded, click
How to do copy & paste:
- Select the above text using mouse, click before the text and drag it down until everything is selected.
- Press Ctrl + C combination to copy.
- Go to Emu8086 source editor and press Ctrl + V combination to paste.
As you may guess, ";" is used for comments, anything after ";" symbol is ignored by compiler.
You should see something like that when program finishes:
Actually the above program writes directly to video memory, so you may see that MOV is a very powerful instruction.
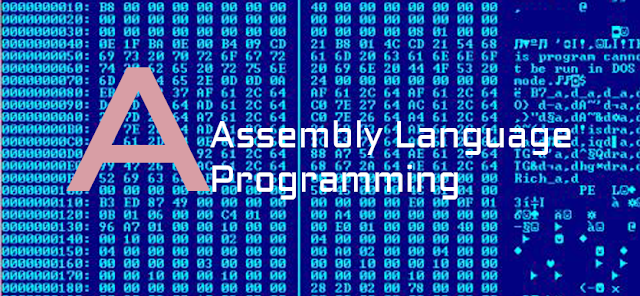
emu8086 is better than NASM, MASM or TASM
Tag: assembly language, assembly instruction, assembly programming, assembly code, assembly guide, emu8086, 8086 microprocessors instruction, instruction sets, instruction sets for 8086, instruction complete set, instruction set complete for 8086, assembly language instruction set, complete 8086 instruction sets microprocessors, complete instruction timing and instruction sets for 8086 microprocessors, 8086 Assembler, TutorialAssembly Language : 8086 Assembler Tutorial Part 12
Assembly Language : 8086 Assembler Tutorial Part 11
Assembly Language : 8086 Assembler Tutorial Part 10
Assembly Language : 8086 Assembler Tutorial Part 9
Assembly Language : 8086 Assembler Tutorial Part 8
Assembly Language : 8086 Assembler Tutorial Part 7
Assembly Language : 8086 Assembler Tutorial Part 6
Assembly Language : 8086 Assembler Tutorial Part 5
Assembly Language : 8086 Assembler Tutorial Part 4
Assembly Language : 8086 Assembler Tutorial Part 3
Assembly Language : 8086 Assembler Tutorial Part 2
Assembly Language : 8086 Assembler Tutorial Part 1
Assembly Language Programming : Complete 8086 instruction sets
Assembly Language Programming : I/O ports - IN/OUT instructions
Assembly Language programming : Emu8086 Assembler Compiling and MASM / TASM compatibility
Assembly Language - string convert - Lowercase , Uppercase
for programming : the language of Number
Assembly Language - Complete Instruction Set and Instruction Timing of 8086 microprocessors
Assembly Language programming : A list of emulator supported interrupts
Assembly Language Programming : Emu8086 Overview, Using Emulator, Virtual Drives
Assembly Language Programming : All about Memory - Global Memory Table and Custom Memory Map
buy me a cup of coffee
My Paypal Account is : ksw.industries@gmail.com
Send me any small amount of money is welcome.buy me a cup of coffee
___________________________________________
Need More Detail ? contact me !!
My Paypal Account is : ksw.industries@gmail.com
buy me a cup of coffeeSend me any small amount of money is welcome.
___________________________________________
Don't know how to send money ? Click here for detail about Paypal account.
About PayPal Payment Methods
What type of PayPal accounts is better.
Don't have money? OK! Here is another way to get the program.
how to get my program - Free of charge
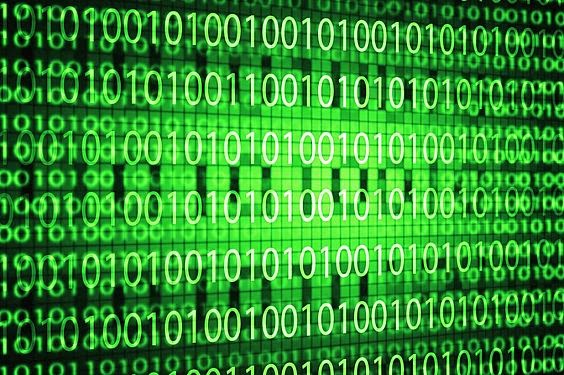
No comments:
Post a Comment