Assembly Language programming : 8086 Assembler Tutorial (Part 9)
The Stack
Stack is an area of memory for keeping temporary data. Stack is used by CALL instruction to keep return address for procedure, RET instruction gets this value from the stack and returns to that offset. Quite the same thing happens when INT instruction calls an interrupt, it stores in stack flag register, code segment and offset. IRET instruction is used to return from interrupt call.
We can also use the stack to keep any other data,
there are two instructions that work with the stack:
PUSH - stores 16 bit value in the stack.
POP - gets 16 bit value from the stack.
Notes:
The stack uses LIFO (Last In First Out) algorithm,
this means that if we push these values one by one into the stack:
1, 2, 3, 4, 5
the first value that we will get on pop will be 5, then 4, 3, 2, and only then 1.
It is very important to do equal number of PUSHs and POPs, otherwise the stack maybe corrupted and it will be impossible to return to operating system. As you already know we use RET instruction to return to operating system, so when program starts there is a return address in stack (generally it's 0000h).
PUSH and POP instruction are especially useful because we don't have too much registers to operate with, so here is a trick:
Here is an example:
Another use of the stack is for exchanging the values,
here is an example:
The exchange happens because stack uses LIFO (Last In First Out) algorithm, so when we push 1212h and then 3434h, on pop we will first get 3434h and only after it 1212h.
The stack memory area is set by SS (Stack Segment) register, and SP (Stack Pointer) register. Generally operating system sets values of these registers on program start.
"PUSH source" instruction does the following:
"POP destination" instruction does the following:
The current address pointed by SS:SP is called the top of the stack.
For COM files stack segment is generally the code segment, and stack pointer is set to value of 0FFFEh. At the address SS:0FFFEh stored a return address for RET instruction that is executed in the end of the program.
You can visually see the stack operation by clicking on [Stack] button on emulator window. The top of the stack is marked with "<" sign.
Assembly Language : 8086 Assembler Tutorial Part 12
Assembly Language : 8086 Assembler Tutorial Part 11
Assembly Language : 8086 Assembler Tutorial Part 10
Assembly Language : 8086 Assembler Tutorial Part 9
Assembly Language : 8086 Assembler Tutorial Part 8
Assembly Language : 8086 Assembler Tutorial Part 7
Assembly Language : 8086 Assembler Tutorial Part 6
Assembly Language : 8086 Assembler Tutorial Part 5
Assembly Language : 8086 Assembler Tutorial Part 4
Assembly Language : 8086 Assembler Tutorial Part 3
Assembly Language : 8086 Assembler Tutorial Part 2
Assembly Language : 8086 Assembler Tutorial Part 1
Assembly Language Programming : Complete 8086 instruction sets
Assembly Language Programming : I/O ports - IN/OUT instructions
Assembly Language programming : Emu8086 Assembler Compiling and MASM / TASM compatibility
Assembly Language - string convert - Lowercase , Uppercase
for programming : the language of Number
Assembly Language - Complete Instruction Set and Instruction Timing of 8086 microprocessors
Assembly Language programming : A list of emulator supported interrupts
Assembly Language Programming : Emu8086 Overview, Using Emulator, Virtual Drives
Assembly Language Programming : All about Memory - Global Memory Table and Custom Memory Map
buy me a cup of coffee
buy me a cup of coffee
Need More Detail ? contact me !!
Send me any small amount of money is welcome.
___________________________________________
Don't know how to send money ? Click here for detail about Paypal account.
About PayPal Payment Methods
What type of PayPal accounts is better.
Don't have money? OK! Here is another way to get the program.
how to get my program - Free of charge
The Stack
Stack is an area of memory for keeping temporary data. Stack is used by CALL instruction to keep return address for procedure, RET instruction gets this value from the stack and returns to that offset. Quite the same thing happens when INT instruction calls an interrupt, it stores in stack flag register, code segment and offset. IRET instruction is used to return from interrupt call.
We can also use the stack to keep any other data,
there are two instructions that work with the stack:
PUSH - stores 16 bit value in the stack.
POP - gets 16 bit value from the stack.
Syntax for PUSH instruction:PUSH REGREG: AX, BX, CX, DX, DI, SI, BP, SP. SREG: DS, ES, SS, CS. memory: [BX], [BX+SI+7], 16 bit variable, etc... immediate: 5, -24, 3Fh, 10001101b, etc... |
Syntax for POP instruction:POP REGREG: AX, BX, CX, DX, DI, SI, BP, SP. SREG: DS, ES, SS, (except CS). memory: [BX], [BX+SI+7], 16 bit variable, etc... |
Notes:
- PUSH and POP work with 16 bit values only!
- Note: PUSH immediate works only on 80186 CPU and later!
The stack uses LIFO (Last In First Out) algorithm,
this means that if we push these values one by one into the stack:
1, 2, 3, 4, 5
the first value that we will get on pop will be 5, then 4, 3, 2, and only then 1.
It is very important to do equal number of PUSHs and POPs, otherwise the stack maybe corrupted and it will be impossible to return to operating system. As you already know we use RET instruction to return to operating system, so when program starts there is a return address in stack (generally it's 0000h).
PUSH and POP instruction are especially useful because we don't have too much registers to operate with, so here is a trick:
- Store original value of the register in stack (using PUSH).
- Use the register for any purpose.
- Restore the original value of the register from stack (using POP).
Here is an example:
ORG 100h
MOV AX, 1234h
PUSH AX ; store value of AX in stack.
MOV AX, 5678h ; modify the AX value.
POP AX ; restore the original value of AX.
RET
END
|
Another use of the stack is for exchanging the values,
here is an example:
ORG 100h
MOV AX, 1212h ; store 1212h in AX.
MOV BX, 3434h ; store 3434h in BX
PUSH AX ; store value of AX in stack.
PUSH BX ; store value of BX in stack.
POP AX ; set AX to original value of BX.
POP BX ; set BX to original value of AX.
RET
END
|
The exchange happens because stack uses LIFO (Last In First Out) algorithm, so when we push 1212h and then 3434h, on pop we will first get 3434h and only after it 1212h.
The stack memory area is set by SS (Stack Segment) register, and SP (Stack Pointer) register. Generally operating system sets values of these registers on program start.
"PUSH source" instruction does the following:
- Subtract 2 from SP register.
- Write the value of source to the address SS:SP.
"POP destination" instruction does the following:
- Write the value at the address SS:SP to destination.
- Add 2 to SP register.
The current address pointed by SS:SP is called the top of the stack.
For COM files stack segment is generally the code segment, and stack pointer is set to value of 0FFFEh. At the address SS:0FFFEh stored a return address for RET instruction that is executed in the end of the program.
You can visually see the stack operation by clicking on [Stack] button on emulator window. The top of the stack is marked with "<" sign.
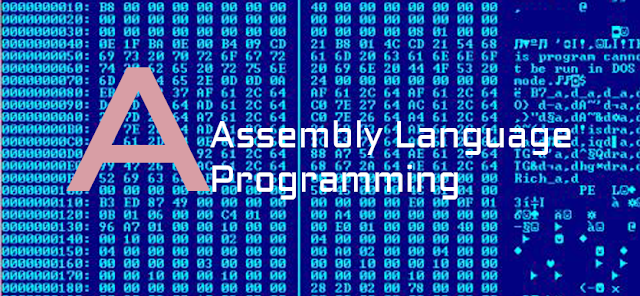
emu8086 is better than NASM, MASM or TASM
Tag: 8086 Assembler, 8086 microprocessors instruction, assembly code, Assembly coding, assembly guide, assembly instruction, assembly language, assembly language instruction set, assembly language programming, Assembly program, assembly programming, capital letter, character convert, complete 8086 instruction sets microprocessors, complete instruction timing and instruction sets for 8086 microprocessors, conversion of characters in assembly language programming 8086, convert, emu8086, instruction complete set, instruction set complete for 8086, instruction sets, instruction sets for 8086, Lower case, Lowercase, print the small character into capital letter, programming 8086 assembly language conversion of small characters to capital, small letter, text string convert, Tutorial,Assembly Language : 8086 Assembler Tutorial Part 12
Assembly Language : 8086 Assembler Tutorial Part 11
Assembly Language : 8086 Assembler Tutorial Part 10
Assembly Language : 8086 Assembler Tutorial Part 9
Assembly Language : 8086 Assembler Tutorial Part 8
Assembly Language : 8086 Assembler Tutorial Part 7
Assembly Language : 8086 Assembler Tutorial Part 6
Assembly Language : 8086 Assembler Tutorial Part 5
Assembly Language : 8086 Assembler Tutorial Part 4
Assembly Language : 8086 Assembler Tutorial Part 3
Assembly Language : 8086 Assembler Tutorial Part 2
Assembly Language : 8086 Assembler Tutorial Part 1
Assembly Language Programming : Complete 8086 instruction sets
Assembly Language Programming : I/O ports - IN/OUT instructions
Assembly Language programming : Emu8086 Assembler Compiling and MASM / TASM compatibility
Assembly Language - string convert - Lowercase , Uppercase
for programming : the language of Number
Assembly Language - Complete Instruction Set and Instruction Timing of 8086 microprocessors
Assembly Language programming : A list of emulator supported interrupts
Assembly Language Programming : Emu8086 Overview, Using Emulator, Virtual Drives
Assembly Language Programming : All about Memory - Global Memory Table and Custom Memory Map
buy me a cup of coffee
My Paypal Account is : ksw.industries@gmail.com
Send me any small amount of money is welcome.buy me a cup of coffee
___________________________________________
Need More Detail ? contact me !!
My Paypal Account is : ksw.industries@gmail.com
buy me a cup of coffeeSend me any small amount of money is welcome.
___________________________________________
Don't know how to send money ? Click here for detail about Paypal account.
About PayPal Payment Methods
What type of PayPal accounts is better.
Don't have money? OK! Here is another way to get the program.
how to get my program - Free of charge
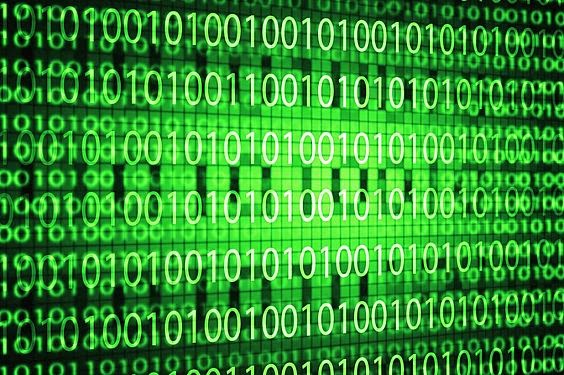
No comments:
Post a Comment